Split Payment by Amount
Split a single payment amount between multiple accounts.
Rapyd enables an employer to pay multiple employees in a single operation by splitting a single payment to multiple Rapyd Wallets. You can divide a payment into 2-10 wallets. Paying directly into wallets is especially useful when your employees do not have bank accounts.
This process can be used to split payments into client wallets, company wallets and personal wallets.
PCI Certification
Only clients with PCI-DSS certification can handle personal identifying information for cards. This method is available to merchants who have signed a special agreement with Rapyd.
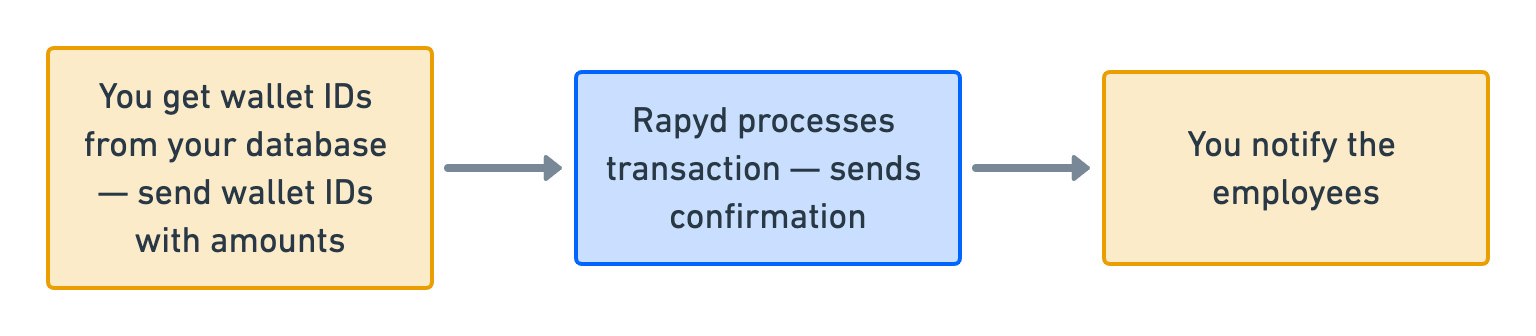
You get the IDs of the employees' wallets from your own database, and send the wallet IDs and the amounts to Rapyd.
Rapyd processes the transaction and sends confirmation to you.
You notify the employees that the payment was made.
Prerequisites
To run the examples of this use case, you must create the following IDs in your own sandbox:
ewallet_ - Run Create Wallet for the wallet of each driver-employee. Use the ID you get in the response. For this use case, you need two separate wallet IDs. For more information, see Creating a Rapyd Wallet.
To send money to the employees' wallets, you ask Rapyd to create a payment. Out of many payment options, you choose to pay from your company's card.
For that, you'll use Create Payment with the following parameters:
Description of Body Parameters
Body Parameter | Description |
---|---|
amount | Enter 300 to pay 300.00 U.S. Dollars. This is the total amount due to all the employees. |
currency | Enter USD as the currency code for U.S. Dollars. |
ewallet | Enter a JSON array of objects. The first object contains the following fields:
The second object contains the following fields:
|
payment_method | Enter an object with the following fields:
|
Create Payment Request
You ask Rapyd to create the payment to the wallets.
Request
// Request URL: POST https://sandboxapi.rapyd.net/v1/payments // Message body: { "amount": 300.00, "currency": "USD", "payment_method": { "type": "us_debit_visa_card", "fields": { "number": "4111111111111111", "expiration_month": "10", "expiration_year": "20", "cvv": "123" } }, "description": "Split payment to multiple wallets", "ewallet": [ { "ewallet": "ewallet_cfb416d9108161347d98acf17f3fd3e8", "amount": 120 }, { "ewallet": "ewallet_4f8009d08b7b41e5d3b356494101d83b", "amount": 180 } ] }
.NET Core
using System; using System.Text.Json; namespace RapydApiRequestSample { class Program { static void Main(string[] args) { try { var requestObj = new { amount = 300.00, currency = "USD", description = "Split payment to multiple wallets", ewallet = new Object[] { new { ewallet = "ewallet_cfb416d9108161347d98acf17f3fd3e8", amount = 120 }, new { ewallet = "ewallet_4f8009d08b7b41e5d3b356494101d83b", amount = 180 }, }, payment_method = new { type = "us_visa_card", fields = new { number = "4111111111111111", expiration_month = "10", expiration_year = "20", cvv = "123", } }, }; string request = JsonSerializer.Serialize(requestObj); string result = RapydApiRequestSample.Utilities.MakeRequest("POST", "/v1/payments", request); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine("Error completing request: " + e.Message); } } } }
JavaScript
const makeRequest = require('<path-to-your-utility-file>/utilities').makeRequest; async function main() { try { const body = { amount: 300, currency: 'USD', payment_method: { type: 'us_visa_card', fields: { number: '4111111111111111', expiration_month: '10', expiration_year: '20', cvv: '123' } }, error_payment_url: 'https://error_example.net', description: 'Split payment to multiple wallets', ewallet: [ { ewallet: 'ewallet_89099803a1e923a3e1fe9fc8f656b425', amount: 70 }, { ewallet: 'ewallet_ecf2ee726c151603968549dd7f1406aa', amount: 30 } ] }; const result = await makeRequest('POST', '/v1/payments', body); console.log(result); } catch (error) { console.error('Error completing request', error); } }
Python
from pprint import pprint from utilities import make_request payment_body = { "amount": 300.00, "currency": "USD", "payment_method": { "type": "us_visa_card", "fields": { "number": "4111111111111111", "expiration_month": "10", "expiration_year": "20", "cvv": "123" } }, "description": "Split payment to multiple wallets", "ewallets": [ { "ewallet": "ewallet_452c8cd682f347b4abee9bbee04eac03", "amount": 120 }, { "ewallet": "ewallet_7af0aeeacbd9bf4d84bf1aeb52f6d8c3", "amount": 180 } ] } create_payment_response = make_request(method='post', path='/v1/payments', body=payment_body) pprint(create_payment_response)
Create Payment Response
Let's take a look at the response.
Response
{ "status": { "error_code": "", "status": "SUCCESS", "message": "", "response_code": "", "operation_id": "81148d4b-185a-4069-9f32-3cf60a579730" }, "data": { "id": "payment_6d8548656ddf97e5c9327c9c46e5498c", "amount": 3000, // ... "currency_code": "USD", // ... "status": "CLO", "description": "Split payment to multiple ewallets", // ... "customer_token": "cus_8a10326d75423c369420f2a2355df353", "payment_method": "card_2ed1da907a0603e3a3c13c737fc073ac", // ... "captured": true, // ... "ewallets": [ { "ewallet_id": "ewallet_cfb416d9108161347d98acf17f3fd3e8", "amount": 120, "percent": 40, "refunded_amount": 0 }, { "ewallet_id": "ewallet_4f8009d08b7b41e5d3b356494101d83b", "amount": 180, "percent": 60, "refunded_amount": 0 } ], // ... "payment_method_type": "us_debit_visa_card", "payment_method_type_category": "card", // ... } }
The data
section of the response shows:
id
- The ID of the 'payment' object.The
original_amount
is 300. This is the total amount paid out.The
currency_code
is USD (U.S. Dollars).The
status
is CLO (closed). This means that the transaction is complete.customer_token
- The ID of the employer's 'customer' object.payment_method
- The ID of the employer's payment method.captured
- The value of true shows that the card payment is completed.ewallets
- The IDs of the employees' wallets, together with the amounts and their respective percentage share of the total payment.payment_method_type
- In this example, the payment method is us_debit_visa_card.payment_method_type_category
- The payment method is a card type.Upon a successful payment Rapyd will send you a Webhook - Payment Confirmation
Now your website can inform the two employees that the transfer to their wallets was successful.