Canceling an Escrow
Cancel a payment being held in escrow.
When a payment is held in escrow, you can determine how long the funds should be held, and also release or cancel the escrow anytime based on your marketplace requirements. This method provides an option to cancel the escrow and immediately release the funds. If there are multiple sellers, the funds are released to all of them.
A customer purchases an item from a seller and an escrow is mistakenly created for this seller, and you decide to cancel it. The money is then released to the seller.
Finding the specific payment methods you'll accept and the corresponding required fields that customers fill out is described under How it Works.
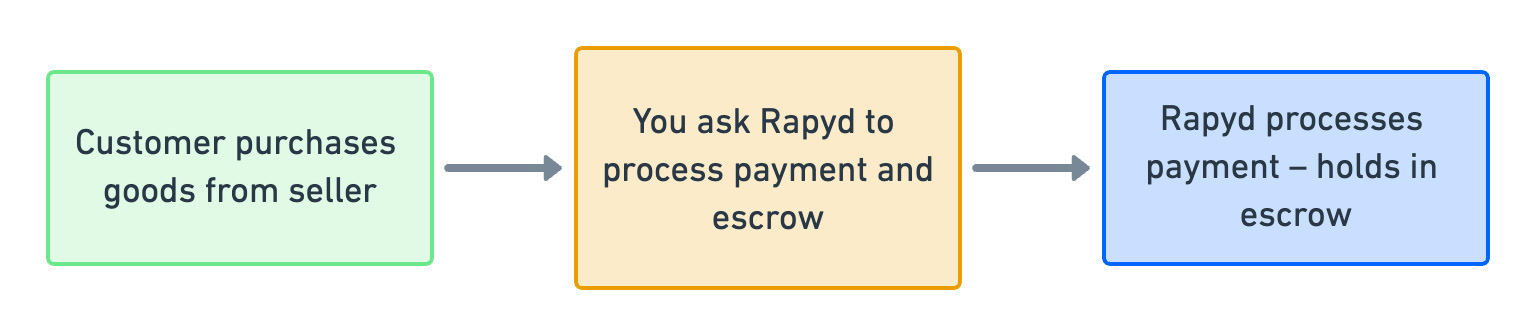
A customer on your marketplace purchases a product from a seller.
The website back end asks Rapyd to process the transaction and hold the funds in escrow for five days.
Rapyd processes the payment and holds the funds in escrow.

Your website back end asks Rapyd to cancel the escrow.
Rapyd cancels the escrow immediately, notifies you that it was canceled successfully, and releases funds to the seller.
Prerequisite
To run the examples of this use case, you must create the following ID in your own sandbox:
ewallet - Run Create wallet for the customer's wallet. Use the 'id' you get in the response.
Decide which payment methods you'll accept. See List Payment Methods by Country.
Find the required fields that customers must fill in for the payment method you chose. See Get Payment Method Required Fields.
The customer bought an item for 501.47 PHP (Philippine Pesos) from a seller on your marketplace website. You ask Rapyd to process the payment and include an escrow for holding the funds for five days.
For that, you'll use Create Payment with the following parameters.
Description of Body Parameters
Body Parameter | Description |
---|---|
amount | Enter 501.47 as the total amount of the payment. |
currency | Enter PHP as the code for Philippine pesos. |
payment_method | Enter a 'payment_method' object that has the following fields:
|
ewallets | In the array, you add one 'ewallet' object that has the following fields:
|
escrow | Enter true to create the escrow. |
escrow_release_days | Enter 5 as the number of days before the escrow funds are automatically released. |
Create Payment Request
You ask Rapyd to process the payment and escrow.
Request
// Request URL: POST https://sandboxapi.rapyd.net/v1/payments // Message body: { "amount": 501.47, "currency": "php", "payment_method": { "type": "ph_dpcard_card" }, "ewallets": [ { "ewallet": "ewallet_090e1ef18c3aa754fd43cce9ee454858", "amount": 501.47 } ], "escrow": true, "escrow_release_days": "5" }
.NET Core
using System; using System.Text.Json; namespace RapydApiRequestSample { class Program { static void Main(string[] args) { try { var requestObj = new { amount = 501.47, currency = "PHP", description = "Payment by card token", payment_method = new { type = "ph_dpcard_card", }, ewallets = new Object[] { new { ewallet = "ewallet_090e1ef18c3aa754fd43cce9ee454858", amount = 501.47 }, }, escrow = true, escrow_release_days = 5 }; string request = JsonSerializer.Serialize(requestObj); string result = RapydApiRequestSample.Utilities.MakeRequest("POST", "/v1/payments", request); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine("Error completing request: " + e.Message); } } } }
Python
from pprint import pprint from utilities import make_request # Create Payment request payment_body = { "amount": 501.47, "currency": "php", "payment_method": { "type": "ph_dpcard_card" }, "ewallets": [ { "ewallet": "ewallet_090e1ef18c3aa754fd43cce9ee454858", "amount": 501.47 } ], "escrow": True, "escrow_release_days": "5" } create_payment_response = make_request(method='post', path='/v1/payments', body=payment_body) pprint(create_payment_response)
Create Payment Response
Let's take a look at the response.
Response
{ "status": { "error_code": "", "status": "SUCCESS", "message": "", "response_code": "", "operation_id": "113ae72c-0456-44d2-bc46-5e00fe52b4f9" }, "data": { "id": "payment_4e1e350df4918b7a5b9d5ba440f6433e", "amount": 0, "original_amount": 501.47, "is_partial": false, "currency_code": "PHP", "country_code": "ph", "status": "ACT", // ... "ewallet_id": "ewallet_090e1ef18c3aa754fd43cce9ee454858", "ewallets": [ { "ewallet_id": "ewallet_090e1ef18c3aa754fd43cce9ee454858", "amount": 501.47, "percent": 100, "released_amount": 0, "refunded_amount": 0 } ], "payment_method_options": {}, "payment_method_type": "ph_dpcard_card", "payment_method_type_category": "bank_redirect", "fx_rate": "", // ... "escrow": { "id": "escrow_933d861296b40ae98f08bec3b8e799ef", "payment": "payment_4e1e350df4918b7a5b9d5ba440f6433e", "amount_on_hold": 0, "total_amount_released": 0, "status": "pending", "escrow_release_days": 5, "created_at": 1580995667, "updated_at": 1580995667, "last_payment_completion": null }, "group_payment": "" } }
The data
section of this response shows that:
The
amount
is 501.47 .The
currency
is PHP.The
ewallets
object indicates:An amount of 501.47 is held in escrow to
ewallet_id
ewallet_090e1ef18c3aa754fd43cce9ee454858 .
The
escrow
object indicates:The escrow ID is escrow_933d861296b40ae98f08bec3b8e799ef.
The payment ID is payment_4e1e350df4918b7a5b9d5ba440f6433e. When you run this example in your own sandbox, you will get a different ID, which you will need for a later step in this use case.
The escrow
status
is pending.The
escrow_release_days
is 5.
You will use the payment ID when you ask Rapyd to cancel the escrow.
The escrow was mistakenly created, and you decide to immediately cancel it. For that, you'll use Update Payment with the following parameters:
Description of Path Parameters
Path Parameter | Description |
---|---|
payment | Enter the payment 'id' that you received when you created the bouquet seller's wallet in your sandbox. For purposes of this use case lesson, we are using payment_4e1e350df4918b7a5b9d5ba440f6433e, which is the payment ID we created in our sandbox. |
Description of Body Parameters
Body Parameter | Description |
---|---|
escrow | Enter false to cancel the escrow. |
Cancel Escrow Request
You ask Rapyd to cancel the escrow.
Request
// Request URL: POST https://sandboxapi.rapyd.net/v1/payments/payment_4e1e350df4918b7a5b9d5ba440f6433e // Message body: { "escrow": false }
.NET Core
using System; using System.Text.Json; namespace RapydApiRequestSample { class Program { static void Main(string[] args) { try { string paymentId = "payment_4e1e350df4918b7a5b9d5ba440f6433e"; var requestObj = new { escrow = false, }; string request = JsonSerializer.Serialize(requestObj); string result = RapydApiRequestSample.Utilities.MakeRequest("POST", $"/v1/payments/{paymentId}", request); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine("Error completing request: " + e.Message); } } } }
JavaScript
const makeRequest = require('<path-to-your-utility-file>/utilities').makeRequest; async function main() { try { const body = { escrow: false }; const result = await makeRequest( 'POST', '/v1/payments/payment_4e1e350df4918b7a5b9d5ba440f6433e', body ); console.log(result); } catch (error) { console.error('Error completing request', error); } }
Python
from pprint import pprint from utilities import make_request cancel_escrow_body = { "escrow": False } cancel_escrow_response = make_request(method='post', path='/v1/payments/payment_4e1e350df4918b7a5b9d5ba440f6433e', body=cancel_escrow_body) pprint(cancel_escrow_response)
Cancel Escrow Response
Let's take a look at the response.
Response
{ "status": { "error_code": "", "status": "SUCCESS", "message": "", "response_code": "", "operation_id": "656b7dd4-7e1e-4f38-b5d9-bb46c4b43ab7" }, "data": { "id": "payment_4e1e350df4918b7a5b9d5ba440f6433e", "amount": 0, "original_amount": 501.47, "is_partial": false, "currency_code": "PHP", "country_code": "ph", "status": "ACT", // ... "created_at": 1580995667, // ... "ewallet_id": "ewallet_090e1ef18c3aa754fd43cce9ee454858", "ewallets": [ { "ewallet_id": "ewallet_090e1ef18c3aa754fd43cce9ee454858", "amount": 501.47, "percent": 100, "released_amount": 0, "refunded_amount": 0 } ], "payment_method_options": {}, "payment_method_type": "ph_dpcard_card", "payment_method_type_category": "bank_redirect", // ... "escrow": { "id": "escrow_933d861296b40ae98f08bec3b8e799ef", "payment": "payment_4e1e350df4918b7a5b9d5ba440f6433e", "amount_on_hold": 0, "total_amount_released": 0, "status": "canceled", "escrow_release_days": 5, "created_at": 1580995667, "updated_at": 1580997283, "last_payment_completion": null }, "group_payment": "" } }
The data
section of this response shows that:
An amount of 501.47 PHP was released to
ewallet_id
ewallet_090e1ef18c3aa754fd43cce9ee454858The
escrow
object indicates:The escrow ID is escrow_933d861296b40ae98f08bec3b8e799ef.
The escrow
status
is canceled.
Looking for More In-Depth Technical Information?
Want to see the Rapyd API methods and objects that you'll use? Visit the Merchant API Reference for more technical details.