Group Payments
Enable up to ten customers to combine their individual payments for a single purchase transaction, or group payment.
Rapyd enables up to ten customers to purchase a product or a service together. This feature is especially useful when customers are sharing the same service. Some use cases may include:
Two or more passengers paying for a ride-sharing service.
Group payment for a bill or service.
PCI Certification
Only clients with PCI-DSS certification can handle personal identifying information for cards. This method is available to merchants who have signed a special agreement with Rapyd.
Finding the specific payment methods you'll accept and the corresponding required fields that customers fill out is described under How it Works.
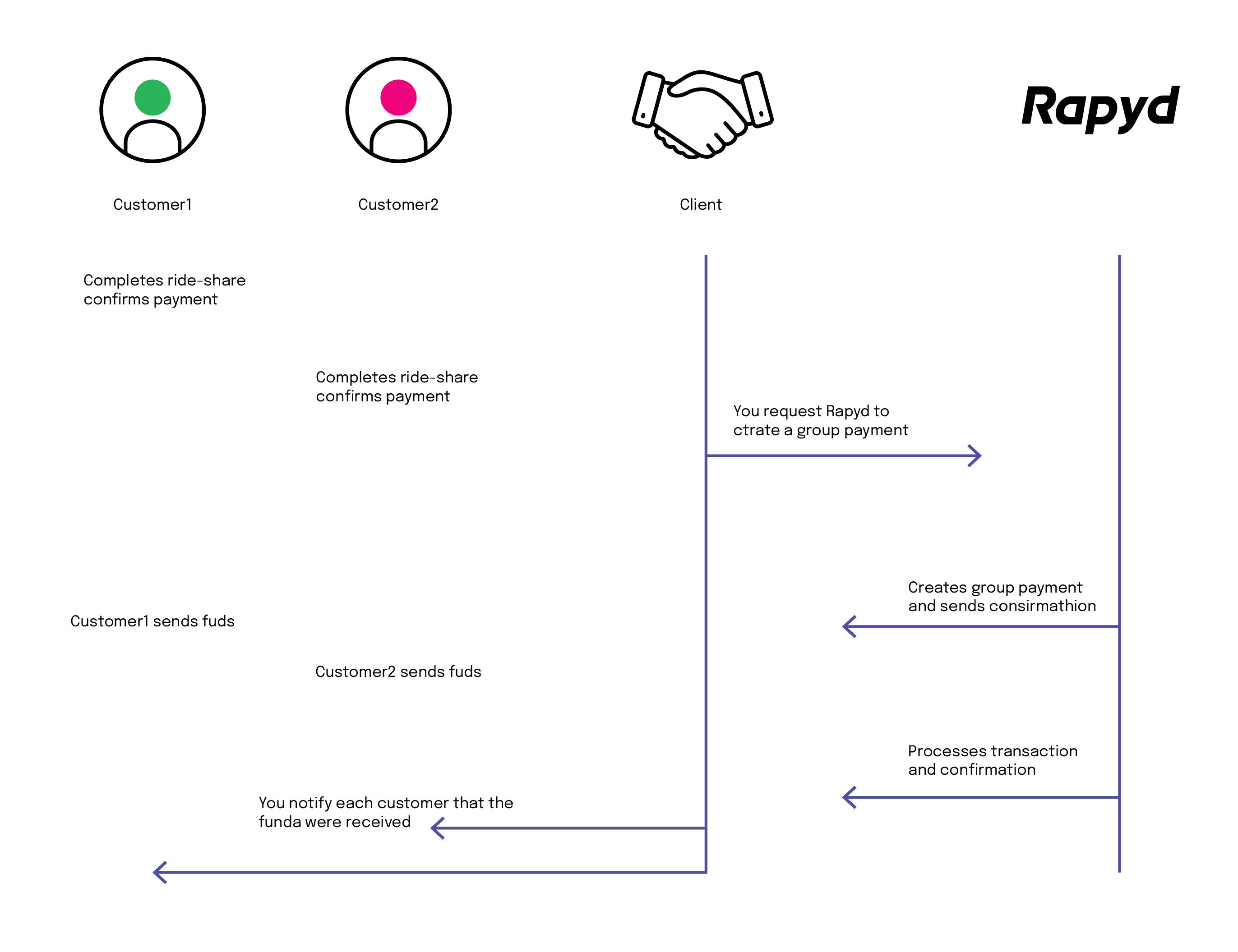
Customer 1 confirms ride-share payment.
Customer 2 ride-share payment.
Client requests Rapyd to create a a group payment.
Rapyd Creates a group payment and sends the confirmation.
Customer 1 sends funds.
Customer 2 sends funds.
Rapyd processes transaction and sends a webhook confirmation.
You notify each customer that the funds were received.
The message sequence diagrams below describe how information is exchanged between Rapyd, the merchant, the merchant's customers, and the card network.
Create Group Payment - Success
Create Group Payment - Failure
Prerequisites
To run the examples of this use case, you must create the following ID in your own sandbox:
ewallet - Run Create Wallet for the wallet of the ride-sharing service. Use the 'id' you get in the response.
Decide which payment methods you'll accept. See List Payment Methods by Country .
Find the required fields that customers must fill in for each payment method. See Get Payment Method Required Fields .
Two customers pay for a ride-sharing service that costs 250 USD (U.S. Dollars). When they arrive at their destination, Test User1 pays 50 USD and Test User2 pays 200 USD.
To transfer the money to the company's wallet, you ask Rapyd to create a group payment. Out of many payment options, both customers choose to pay by bank transfers.
For that, you'll use Create Group Payment with the following parameters:
Description of Body Parameters
Body Parameter | Description |
---|---|
payments | Enter a list of First
Second
|
Create Group Payment Request
You ask Rapyd to create the group payment to your company's wallet.
Request
// Request URL: POST https://sandboxapi.rapyd.net/v1/payments/group_payments // Message body: { "merchant_reference_id": "12345689", "payments": [ { "payment_method": { "type": "us_debit_discover_card", "fields": { "number": "4111111111111111", "expiration_month": "11", "expiration_year": "21", "cvv": "123", "name": "Test User1", "address": "123 Main street, Anytown" }, "metadata": null }, "amount": 50, "currency": "USD", "ewallets": [{ "ewallet": "ewallet_4f8009d08b7b41e5d3b356494101d83b" } ] }, { "payment_method": { "type": "us_mastercard_card", "fields": { "number": "4111111111111111", "expiration_month": "11", "expiration_year": "21", "cvv": "123", "name": "Test User2", "address": "123 Main street, Anytown" }, "metadata": null }, "amount": 200, "currency": "USD", "ewallets": [{ "ewallet": "ewallet_4f8009d08b7b41e5d3b356494101d83b" } ] } ] }
.NET Core
using System; using System.Text.Json; namespace RapydApiRequestSample { class Program { static void Main(string[] args) { try { var payment_a = new { payment_method = new { type = "us_discover_card", fields = new { number = "4111111111111111", expiration_month = "11", expiration_year = "21", cvv = "123", name = "John Doe", address = "123 Main Street, Anytown, NY" } }, amount = 50, currency = "USD", ewallets = new Object[] { new { ewallet = "ewallet_4f8009d08b7b41e5d3b356494101d83b" } } }; var payment_b = new { payment_method = new { type = "us_mastercard_card", fields = new { number = "4111111111111111", expiration_month = "11", expiration_year = "21", cvv = "123", name = "John Doe", address = "123 Main Street, Anytown, NY" } }, amount = 200, currency = "USD", ewallets = new Object[] { new { ewallet = "ewallet_4f8009d08b7b41e5d3b356494101d83b" } } }; var requestObj = new { payments = new Object[] { payment_b, payment_a }, merchant_reference_id = "12345689" }; string request = JsonSerializer.Serialize(requestObj); string result = RapydApiRequestSample.Utilities.MakeRequest("POST", "/v1/payments/group_payments", request); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine("Error completing request: " + e.Message); } } } }
JavaScript
const makeRequest = require('<path-to-your-utility-file>/utilities').makeRequest; async function main() { try { const body = { merchant_reference_id: '12345689', payments: [ { payment_method: { type: 'us_discover_card', fields: { number: '4111111111111111', expiration_month: '11', expiration_year: '21', cvv: '123', name: 'Jane Doe', address: '123 Main Street, Anytown' } }, amount: 50, currency: 'USD', ewallets: [ { ewallet: 'ewallet_4f8009d08b7b41e5d3b356494101d83b' } ] }, { payment_method: { type: 'us_mastercard_card', fields: { number: '4111111111111111', expiration_month: '11', expiration_year: '21', cvv: '123', name: 'John Doe', address: '123 Main Street, Anytown' } }, amount: 200, currency: 'USD', ewallets: [ { ewallet: 'ewallet_4f8009d08b7b41e5d3b356494101d83b' } ] } ] }; const result = await makeRequest('POST', '/v1/payments/group_payments', body); console.log(result); } catch (error) { console.error('Error completing request', error); } }
PHP
<?php $path = $_SERVER['DOCUMENT_ROOT']; $path .= "/<path-to-your-utility-file>/utilities.php"; include($path); $body = [ "merchant_reference_id" => "12345689", "payments" => [ [ "payment_method" => [ "type" => "us_discover_card", "fields" => [ "number" => "4111111111111111", "expiration_month" => "11", "expiration_year" => "21", "cvv" => "123", "name" => "Jane Doe", "address" => "123 Main street, Anytown" ] ], "amount" => 50, "currency" => "USD", "ewallets" => [[ "ewallet" => "ewallet_4f8009d08b7b41e5d3b356494101d83b" ] ] ], [ "payment_method" => [ "type" => "us_mastercard_card", "fields" => [ "number" => "4111111111111111", "expiration_month" => "11", "expiration_year" => "21", "cvv" => "123", "name" => "John Doe", "address" => "123 Main street, Anytown" ] ], "amount" => 200, "currency" => "USD", "ewallets" => [[ "ewallet" => "ewallet_4f8009d08b7b41e5d3b356494101d83b" ] ] ] ] ]; try { $object = make_request('post', '/v1/payments/group_payments', $body); var_dump($object); } catch (Exception $e) { echo "Error: $e"; } ?>
Python
from pprint import pprint from utilities import make_request group_payment_body = { "merchant_reference_id": "12345689", "payments": [{ "payment_method": { "type": "us_discover_card", "fields": { "number": "4111111111111111", "expiration_month": "11", "expiration_year": "21", "cvv": "123", "name": "Jane Doe", "address": "123 Main street, Anytown" } }, "amount": 50, "currency": "USD", "ewallets": [{ "ewallet": "ewallet_4f8009d08b7b41e5d3b356494101d83b" }] }, { "payment_method": { "type": "us_mastercard_card", "fields": { "number": "4111111111111111", "expiration_month": "11", "expiration_year": "21", "cvv": "123", "name": "John Doe", "address": "123 Main street, Anytown" } }, "amount": 200, "currency": "USD", "ewallets": [{ "ewallet": "ewallet_4f8009d08b7b41e5d3b356494101d83b" }] }] } create_group_payment_response = make_request(method='post', path='/v1/payments/group_payments', body=group_payment_body) pprint(create_group_payment_response)
Get Group Payment Response
Let's take a look at the response.
Response
{ "status": { "error_code": "", "status": "SUCCESS", "message": "", "response_code": "", "operation_id": "04d56aea-edee-4aa6-aea2-9d8ab7df98b6" }, "data": { "id": "gp_b323dd9cf3e6a97f4cbca1d223e828b6", "amount": 250, "amount_to_replace": 0, "status": "closed", "currency": "USD", "country": "US", "merchant_reference_id": "12345689", "description": "", "metadata": { "user_defined": "silver" }, "expiration": null, "cancel_reason": null, "payments": [ { "id": "payment_a2e52670fd0d59ce6f4c7520fdd8bdc1", "amount": 50, "original_amount": 50, "is_partial": false, "currency_code": "USD", "country_code": "US", "status": "CLO", // ... "paid": true, "paid_at": 1581435110, // ... "ewallet_id": "ewallet_4f8009d08b7b41e5d3b356494101d83b", "ewallets": [ { "ewallet_id": "ewallet_4f8009d08b7b41e5d3b356494101d83b", "amount": 50, "percent": 100, "refunded_amount": 0 } ], "payment_method_options": {}, "payment_method_type": "us_debit_discover_card", "payment_method_type_category": "card", "fx_rate": "", "merchant_requested_currency": null, "merchant_requested_amount": null, "fixed_side": "", "payment_fees": null, "invoice": "", "escrow": null, "group_payment": "gp_b323dd9cf3e6a97f4cbca1d223e828b6" }, { "id": "payment_4dc0e0ad8590ac86157478fd1f678c19", "amount": 200, "original_amount": 200, "is_partial": false, "currency_code": "USD", "country_code": "US", "status": "CLO", // ... "paid": true, "paid_at": 1581435110, // ... "ewallet_id": "ewallet_4f8009d08b7b41e5d3b356494101d83b", "ewallets": [ { "ewallet_id": "ewallet_4f8009d08b7b41e5d3b356494101d83b", "amount": 200, "percent": 100, "refunded_amount": 0 } ], "payment_method_options": {}, "payment_method_type": "us_mastercard_card", "payment_method_type_category": "card", "fx_rate": "", "merchant_requested_currency": null, "merchant_requested_amount": null, "fixed_side": "", "payment_fees": null, "invoice": "", "escrow": null, "group_payment": "gp_b323dd9cf3e6a97f4cbca1d223e828b6" } ] } }
The data
section of the response shows:
id
- The ID of thegroup_payment
is gp_b323dd9cf3e6a97f4cbca1d223e828b6. Agroup_payment
ID indicates that the payment was funded by multiple customers.There are two payment IDs. When you run this example in your own sandbox, you will get different IDs, which you will need for later steps in this use case.
payment_a2e52670fd0d59ce6f4c7520fdd8bdc1 is the ID of Jane Doe's payment.
payment_4dc0e0ad8590ac86157478fd1f678c19 is the ID of John Doe's payment.
The
currency_code
is USD (U.S. Dollars).The
status
is ACT (Active and awaiting payment).customer_token
- There are two customer tokens:cus_e0f030467d787e3e3e4570e3e623426a is the customer ID of Jane Doe.
cus_f72b1a5d07af0c7aa9ff48008697456c is the customer ID of John Doe.
ewallets
- The ID of your company eWallet in both payments. The eWallet ID is ewallet_2dae0b1fea197d342868ab127c35592d and the amount is 200 USD.payment_method_type
- There are two payment methods:us_debit_discover_card is the ID of Jane Doe's payment.
us_mastercard_card is the payment method of John Doe's payment.
payment_method_type_category
- The payment method is a card type for both payments.
Your website or app notifies the customers to transfer the funds by bank transfer. When the transfers are made by both John and Jane Doe, Rapyd processes the transaction and sends a Group Payment Completed webhook confirming that the payment has been made.
Simulating a Bank Transfer
The sandbox does not directly simulate a bank transfer. You can simulate this action with the procedure described in Complete Payment. For this, you will need the payment ID that you received for each customer in the response to 'Create Group Payment'.
Configure your system to receive webhooks with the procedure described in Defining a Webhook Endpoint.
Now your website or app can inform the two customers that the transfers to your client wallet were successful.