Bank Transfers
Move funds between accounts at different banks.
A bank transfer is a payment submission from your customer's bank account to you. Many customers rely on bank transfers as their primary payment method. Rapyd makes it easy for your customers to use bank transfers to move funds between accounts and pay for purchases. With bank transfers you can make the following types of payments:
The bank transfer process below explains how to:
Add bank transfer payments to your business workflow.
Create a bank transfer payment request.
Capture the payment response.
Bank Transfer Checkout
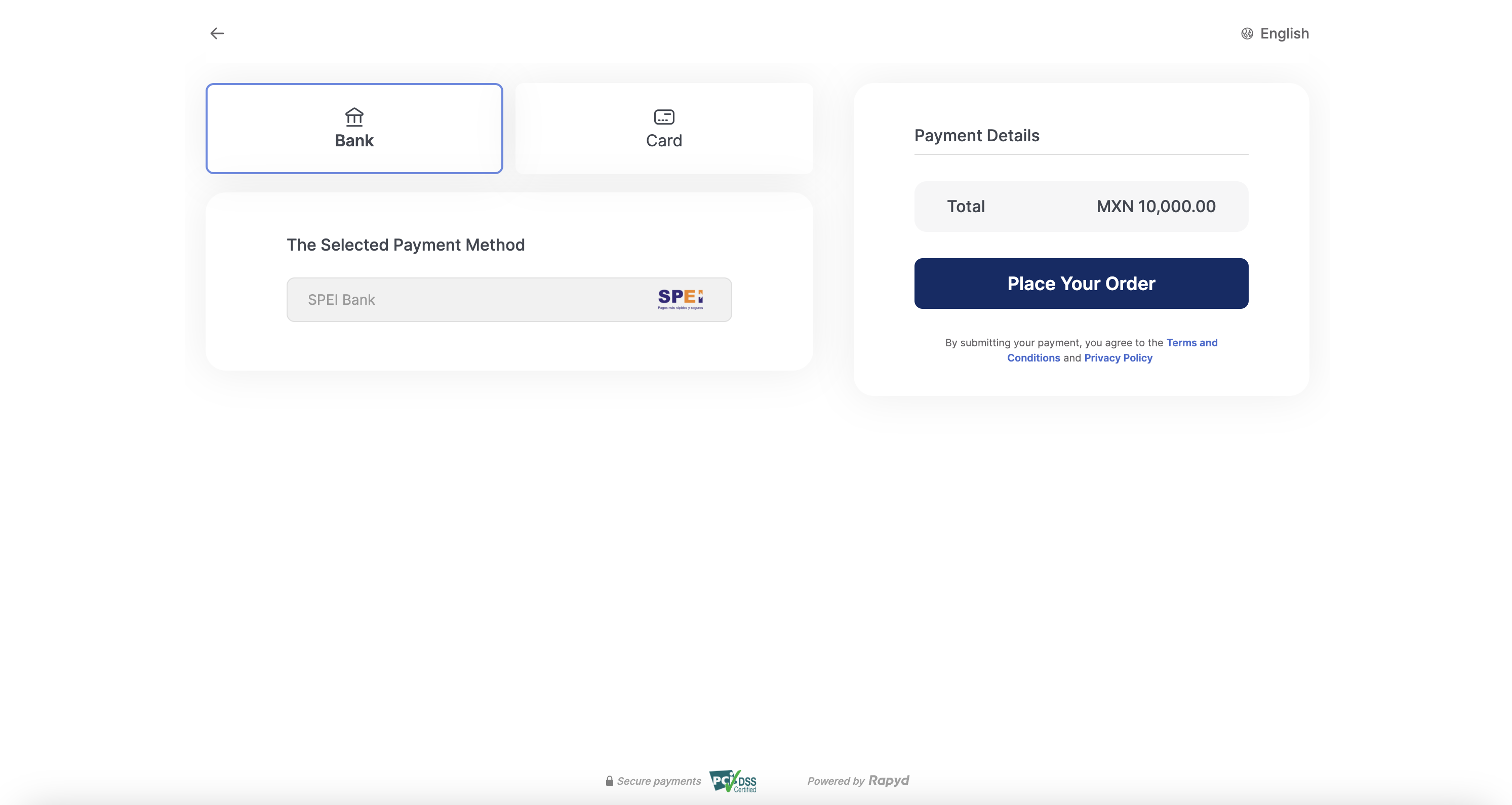
Finding the specific bank transfer payment methods you'll accept and the corresponding required fields that customers fill out is described under How it Works.

Customer confirms a bank transfer payment in your checkout cart.
Your website back end requests Rapyd to process a bank transfer payment.
Rapyd processes the transaction and responds with payment transaction details.
The response includes payment instructions for the customer.
Your website displays the instructions to the customer.

The customer contacts the bank (for example, using the bank's website, mobile app, phone support, or branch) and directs the bank to transfer the money.
The customer's bank transfers the money.

Rapyd is notified that the transfer was successful.
Rapyd notifies your website back end through a Payment Completed Webhook that the payment was completed successfully.
You notify the customer that the purchase succeeded.
The message sequence diagrams below describe how information is exchanged between Rapyd, the merchant, the merchant's customers, and the bank.
Create Bank Transfer Payment - Success
Create Bank Transfer Payment - Failure
Create Bank Transfer Payment - Transaction Expired
Decide the bank transfer payment types you'll accept in a chosen country, so your customer can specify their payment methods on your payment page.
For illustration purposes, we will use MX (Mexico) and MXN (Mexican Peso) for country and currency in the sample codes below. The payment type is MX SPEI Bank Transfer.
To do that, use List Payment Methods by Country with the following parameters:
Description of Query Parameters
Query Parameter | Description |
---|---|
country | Enter MX as the country code for Mexico. |
currency | Enter MXN as the currency code for Mexican pesos. |
List Payment Methods by Country Request
Request for a list of all available payment methods.
Request
// Request URL: GET https://sandboxapi.rapyd.net/v1/payment_methods/country?country=MX¤cy=MXN // Message body absent
.NET Core
using System; namespace RapydApiRequestSample { class Program { static void Main(string[] args) { try { string country = "MX"; string currency = "MXN"; string result = RapydApiRequestSample.Utilities.MakeRequest("GET", $"/v1/payment_methods/country?country={country}¤cy={currency}"); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine("Error completing request: " + e.Message); } } } }
JavaScript
const makeRequest = require('<path-to-your-utility-file>/utilities').makeRequest; async function main() { try { const result = await makeRequest('GET', '/v1/payment_methods/country?country=MX¤cy=MXN'); console.log(result); } catch (error) { console.error('Error completing request', error); } }
PHP
<?php $path = $_SERVER['DOCUMENT_ROOT']; $path .= "/<path-to-your-utility-file>/utilities.php"; include($path); try { $object = make_request('get', '/v1/payment_methods/country?country=MX¤cy=MXN'); var_dump($object); } catch (Exception $e) { echo "Error: $e"; } ?>
Python
from pprint import pprint from utilities import make_request country = 'MX' currency = 'MXN' results = make_request(method='get', path=f'/v1/payment_methods/country?country={country}¤cy={currency}') pprint(results)
List Payment Methods by Country Response
List Payment Methods by Country describes the fields in the response.
Response
{ "status": { "error_code": "", "status": "SUCCESS", "message": "", "response_code": "", "operation_id": "8065d391-d324-41cb-8b36-a7d97bd0ed6c" }, "data": [{ "type": "mx_spei_bank", "name": "SPEI Bank", "category": "bank_transfer", "image": "https://iconslib.rapyd.net/checkout/mx_spei_bank.png", "country": "mx", "payment_flow_type": "bank_transfer", "currencies": [ "MXN" ], "status": 1, "is_cancelable": false, "payment_options": [{ "name": "customer", "type": "customer", "regex": "", "description": "make sure a customer was created with first_name, last_name, email and address", "is_required": true, "is_updatable": false }, { "name": "ewallet", "type": "string", "regex": "", "description": "make sure to pass an ewallet", "is_required": false, "is_updatable": false } ], "is_expirable": false, "is_online": false, "minimum_expiration_seconds": null, "maximum_expiration_seconds": null } ] }
The data
section of this response shows that mx_spei_bank is an acceptable payment method for bank transfers.
Note
A full API response usually lists many payment methods.
Decide the fields that the customer must complete for the payment method, by using Get Payment Method Required Fields with the following parameter:
Description of Path Parameters
Path Parameter | Description |
---|---|
type | Enter mx_spei_bank as the payment method type. |
Get Payment Method Required Fields Request
Request a set of required fields for an mx_spei_bank payment.
Request
// Request URL: GET https://sandboxapi.rapyd.net/v1/payment_methods/mx_spei_bank/required_fields // Message body absent
.NET Core
using System; namespace RapydApiRequestSample { class Program { static void Main(string[] args) { try { string type = "mx_spei_bank"; string result = RapydApiRequestSample.Utilities.MakeRequest("GET", $"/v1/payment_methods/{type}/required_fields"); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine("Error completing request: " + e.Message); } } } }
JavaScript
const makeRequest = require('<path-to-your-utility-file>/utilities').makeRequest; async function main() { try { const result = await makeRequest('GET', '/v1/payment_methods/mx_spei_bank/required_fields'); console.log(result); } catch (error) { console.error('Error completing request', error); } }
PHP
<?php $path = $_SERVER['DOCUMENT_ROOT']; $path .= "/<path-to-your-utility-file>/utilities.php"; include($path); try { $object = make_request('get', '/v1/payment_methods/mx_spei_bank/required_fields'); var_dump($object); } catch (Exception $e) { echo "Error: $e"; } ?>
Python
from pprint import pprint from utilities import make_request payment_method = 'mx_spei_bank' results = make_request(method='get', path=f'/v1/payment_methods/{payment_method}/required_fields') pprint(results)
Get Payment Method Required Fields Response
Get Payment Method Required Fields describes the fields in the response.
Response
{ "status": { "error_code": "", "status": "SUCCESS", "message": "", "response_code": "", "operation_id": "19ea2c3f-7e09-4834-bd9f-3415109631ab" }, "data": { "type": "mx_spei_bank", "fields": [ { "name": "amount", "type": "number", "regex": "[0-9]*.\\\\\\\\.[0-9]{2}", "description": "Must have two decimal places.", "is_required": false, "is_updatable": false }, { "name": "description", "type": "string", "regex": "[a-zA-z]{0,49}", "description": "Description of the payment transaction", "is_required": false, "is_updatable": false }, { "name": "expiration", "type": "number", "regex": "", "description": "End of the time allowed for customer to make this payment, in Unix time.", "is_required": false, "is_updatable": false }, { "name": "metadata", "type": "object", "regex": "", "description": "A JSON object defined by the Rapyd partner.", "is_required": false, "is_updatable": false } ], "payment_method_options": [], "payment_options": [ { "name": "customer", "type": "customer", "regex": "", "description": "make sure a customer was created with first_name, last_name, email and address", "is_required": true, "is_updatable": false }, { "name": "ewallet", "type": "string", "regex": "", "description": "make sure to pass an ewallet", "is_required": false, "is_updatable": false } ], "minimum_expiration_seconds": null, "maximum_expiration_seconds": null } }
When your customer checks out on your website, use Create Payment with the following parameters to get Rapyd to process your customer's bank transfer payment:
Description of Body Parameters
Body Parameter | Description |
---|---|
amount | Enter 10,000 as the payment amount. |
currency | Enter MXN as the currency code for Mexican pesos. |
payment_method | Enter an object with the following field: |
Create Payment Request
Request Rapyd to process Jane's payment of 10,000 MXN (Mexican Pesos) as a bank transfer, as shown in the request below:
Request
// Request URL: POST https://sandboxapi.rapyd.net/v1/payments // Message body: { "amount": 100000, "currency": "MXN", "payment_method": { "type": "mx_spei_bank" } }/ Req // Message body absent
.NET Core
using System; using System.Text.Json; namespace RapydApiRequestSample { class Program { static void Main(string[] args) { try { var requestObj = new { amount = 100, currency = "MXN", payment_method = new { type = "mx_spei_bank", } }; string request = JsonSerializer.Serialize(requestObj); string result = RapydApiRequestSample.Utilities.MakeRequest("POST", "/v1/payments", request); Console.WriteLine(result); } catch (Exception e) { Console.WriteLine("Error completing request: " + e.Message); } } } }
JavaScript
const makeRequest = require('<path-to-your-utility-file>/utilities').makeRequest; async function main() { try { const body = { amount: 100, currency: 'MXN', payment_method: { type: 'mx_spei_bank' } }; const result = await makeRequest('POST', '/v1/payments', body); console.log(result); } catch (error) { console.error('Error completing request', error); } }
PHP
<?php $path = $_SERVER['DOCUMENT_ROOT']; $path .= "/<path-to-your-utility-file>/utilities.php"; include($path); $body = [ "amount" => "100", "currency" => "MXN", "payment_method" => [ "type" => "mx_spei_bank" ] ]; try { $object = make_request('post', '/v1/payments', $body); var_dump($object); } catch (Exception $e) { echo "Error: $e"; } ?>
Python
from pprint import pprint from utilities import make_request payment_body = { "amount": 100, "currency": "MXN", "payment_method": { "type": "mx_spei_bank" } } create_payment_response = make_request(method='post', path='/v1/payments', body=payment_body) pprint(create_payment_response)
Create Payment Response
The response is generated below:
See Create Payment for a description of the fields in the response.
CLABE
As of June 1, 2004, Mexico has used CLABE for numbering bank accounts, this standard is required for sending and receiving domestic inter-bank electronic fund transfers.
Response
{ "status": { "error_code": "", "status": "SUCCESS", "message": "", "response_code": "", "operation_id": "0993fb79-2c41-4b41-998b-5101189db34f" }, "data": { "id": "payment_d4fbfe943226bdd14ff35bf3f5d39107", "amount": 0, "original_amount": 100, "is_partial": false, "currency_code": "MXN", "country_code": "mx", "status": "ACT", // ... "customer_token": "cus_b4d4d442f32ae24ed9616753573ea755", "payment_method": "other_52f165b04eb609f40559f612ed683245", // ... "created_at": 1594033690, // ... "visual_codes": { "payCode": "888455799438564343" }, "textual_codes": {}, "instructions": [ { "name": "instructions", "steps": [ { "step1": "Direct the end-user to make payment to the CLABE provided." } ] } ], "ewallet_id": "ewallet_903e0e470af2d76a45dab68f62cd23b5", "ewallets": [ { "ewallet_id": "ewallet_903e0e470af2d76a45dab68f62cd23b5", "amount": 10000, "percent": 100, "refunded_amount": 0 } ], "payment_method_options": {}, "payment_method_type": "mx_spei_bank", "payment_method_type_category": "bank_transfer", // ... } }
The data
section of this response shows:
The payment
id
is payment_d4fbfe943226bdd14ff35bf3f5d39107.
Note
When you run this in your own sandbox, you will get a different ID, which you will need for a later step.
The
original_amount
is 100,000.The
currency_code
is MXN (Mexican pesos).The
status
is ACT.
Payment Completion
This means that the payment process is active and awaiting payment. Instructions for your customer to complete the payment.
Your website will provide these instructions to your customer.
After your customer successfully pays and Rapyd is informed that the money was received, Rapyd sends you a webhook with the details of the transaction.
Simulating a Bank Transfer
The sandbox does not directly simulate a bank transfer. You can simulate this action with the procedure described in Complete Payment. For this, you will need the payment ID that you generated in your sandbox.
Configure your system to receive webhooks with the procedure described in Defining a Webhook Endpoint.
Let's take a look at Webhook - Payment Completed.
Webhook
{ "id": "wh_7f18c2b90892652fe7ff2cb7d0537732", "type": "PAYMENT_COMPLETED", "data": { "id": "payment_d4fbfe943226bdd14ff35bf3f5d39107", "fee": 0, "paid": true, "order": null, "amount": 10000, "status": "CLO", // ... "captured": true, // ... "created_at": "1551173291", // ... "instructions": [ { "name": "instructions", "steps": [ { "step1": "Direct the end-user to make payment to the CLABE provided." } ] } ], // ... "currency_code": "MXN", // ... "customer_token": "cus_b4d4d442f32ae24ed9616753573ea755", "payment_method": "other_52f165b04eb609f40559f612ed683245", // ... "original_amount": 10000, // ... "payment_method_type_category": "bank_transfer" }, // ... "status": "CLO", "created_at": 1594033690 }
The data
section of the webhook contains the same type of information as the response to the Create Payment request, except that the status now appears as CLO (closed). This shows that the payment is complete.
At this point, you can display a (success) status on the screen to let the customer know that their purchase was successful and that they can go ahead and collect their purchased items.